IntelliSense-like autocomplete for Vim with clang
Contents
Note: This article is a bit focused on Mac OS X (Snow Leopard). I've not tested this on Windows or Linux. My feeling is that on Linux the same principles apply, while on Windows a bit of extra work is required.
Introduction
Traditionally the Vim autocompletion <C-n> works very good for normal text, but it's not context aware in programming languages, e. g. it fails in situations like this:
The editor has to understand the language for accurately completing the code. While the situation got better with Vim 7 and its omni-completion, previously I was unable to get a precise autocompletion on a level with IDEs like Microsoft Visual Studio.
So then I've installed the clang_complete plugin and I was blown away by its autocompletion quality for C/C++ code (sorry, no Java or other languages). The trick is that the clang_complete plugin is using clang to actually compile the code and then use the compiler information for autocompletion. That means in theory clang_complete knows what the compiler knows. Also there is no need to create a tags file for your project or included stuff like STL. The plugin is always up to date, which is a huge advantage.
Three plugins are relevant here:- clang_complete
- SuperTab (optional)
- snipMate (optional)
SuperTab maps the <Tab> key to autocomplete, and automatically decides which Vim completion mode is used. So you don't have to think about the different completion key combinations (i. e. text completion <C-n>, omnicomplete <C-x>+<C-o> or user function <C-x>+<C-u>). Just use <Tab>.
The snipMate plugin is on the list, because it is a) very useful and b) needs a little adjustment to play well with the other two plugins (see the Setup section). With snipMate you can press <Tab> to insert code snippets depending on your current file type. For example you type:
{
/* code */
}
Setup
First of all, you need clang (a C language family frontend for LLVM) itself installed. Mac OS X (Snow Leopard) already ships with clang. The clang_complete wiki page has an overview on how to install clang on different systems:
https://github.com/Rip-Rip/clang_complete/wiki
Then you need the Vim plugins. I recommend to use their respective
Github page to download them, but you can also download them from
vim.org, though the versions from vim.org might lack a bit behind. It
has to be noted that clang_complete does not work together with
OmniCppComplete. So if you currently use this plugin, remove it.
Follow the installation instructions for each plugin, but basically the
installation is copying the plugin files to your .vim folder (or
vimfiles on Windows).
https://github.com/Rip-Rip/clang_complete
SuperTab:
https://github.com/ervandew/supertab
snipMate:
https://github.com/msanders/snipmate.vim
I have mentioned that snipMate needs a bit of adjustment to play well with the other plugins. The thing is that snipMate has a snippet pattern, that turns a dot into brackets []. Which is bad when you type a dot and then hit <Tab> to autocomplete with SuperTab.
[${1}]${2}
# [${1}]${2}
Apart from that, the plugins are aware of each other and play well together. I have added a few settings to my .vimrc to optimize things a bit:
" Complete options (disable preview scratch window) set completeopt = menu,menuone,longest " Limit popup menu height set pumheight = 15 " SuperTab option for context aware completion let g:SuperTabDefaultCompletionType = "context" " Disable auto popup, use <Tab> to autocomplete let g:clang_complete_auto = 0 " Show clang errors in the quickfix window let g:clang_complete_copen = 1
If clang fails to compile your code, you won't get autocompletion. In some cases (e. g. missing header path for clang) it can help to create a .clang_complete file and add additional compiler parameters to it. See the clang_complete documentation for details.
Demonstration
My example project here is my 3-D engine. While it's not a super big project, it can confuse other Vim autocompletion systems. Let's take a look at line 65 of the following source code, which is actually a bit convoluted, but great to test the autocompletion system.
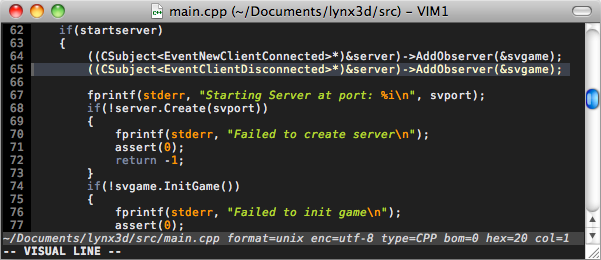
We have a template class for the observer pattern, pointers, casts and calls to member functions. Let's see how clang_complete behaves if we try to rewrite the second call to the AddObserver method:

And the clang_complete autocompletion plugin has no trouble with this construction and gives accurate results. I think this is amazing!
Animated demonstration (click to start or restart the animation):
Appendix: STL
Even the Standard Template Library (STL) works right out of the box with clang_complete. Here are two examples.
Working with an iterator:
Diplaying the members of a std::string object:

The colorscheme used in the screenshots is Mustang
and the font is DejaVu Sans Mono on MacVim 7.3.
If you have any questions or suggestions, send me a mail: